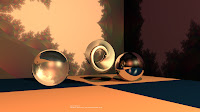
__device__ void
mandelbrotSet( const SceneInfo& sceneInfo, const float x, const float y,
float4& color )
{
float W = (float)gTextureWidth;
float H = (float)gTextureHeight;
float MinRe = -2.f;
float MaxRe = 1.f;
float MinIm = -1.2f;
float MaxIm = MinIm
+ (MaxRe - MinRe) * H/W;
float
Re_factor = (MaxRe - MinRe) / (W - 1.f);
double Im_factor = (MaxIm - MinIm) / (H - 1.f);
float
MaxIterations = 20.f+sceneInfo.pathTracingIteration.x;
float c_im = MaxIm - y*Im_factor;
float c_re =
MinRe + x*Re_factor;
float Z_re = c_re;
float Z_im = c_im;
bool isInside = true;
unsigned n;
for( n = 0; isInside && n < MaxIterations;
++n )
{
float Z_re2 = Z_re*Z_re;
float Z_im2 = Z_im*Z_im;
if ( Z_re2+Z_im2>4.f )
{
isInside = false;
}
Z_im = 2.f*Z_re*Z_im+c_im;
Z_re = Z_re2 - Z_im2+c_re;
}
color.x += Z_re/64.f;
color.y += Z_im/64.f;
color
*= (n/MaxIterations);
}
GPUs amaze me for making it possible to enjoy real-time computation of ray-traced images, with the Mandelbrot set... as a texture.